The Lorenz Attractor
For drawing the Lorenz attractor,
or coping with a similar situation.
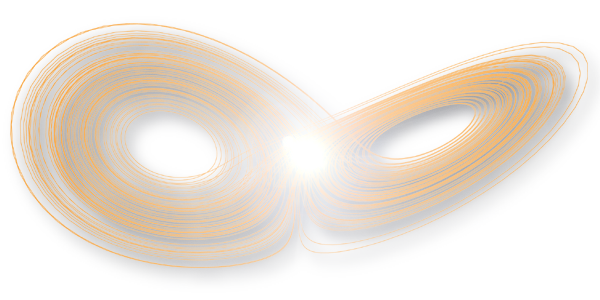
Source code
l1.c
#include <stdio.h>
#include <stdlib.h>
#include "rk.h"
#include "lorenz.h"
#define N 3
#define h 0.01
#define T 10000
main()
{
int t, i;
double *X0 = vector(N),*X1 = vector(N);
X0[0] = 10.0;
X0[1] = 20.0;
X0[2] = 30.0;
for(t = 0; t < = T - 1; t++)
{
for(i = 0; i < = N - 1; i++)
{
printf("%f", X0[i]);
if(i == N - 1) putchar('\n');
else putchar(' ');
}
rk(h,N, lorenz, h*t, X0, X1);
copy_vector(N, X1, X0);
}
return 0;
}
rk.h
#include <stdlib.h>
double *vector(int N)
{
return (double *)malloc(N * sizeof(double));
}
void free_vector(double *v) Releasing vector region
{
p
free(v);
}
void copy_vector(int N, double a[], double b[]) Copying vectors
{
int i;
for(i = 0; i <= N - 1; i++) b[i] = a[i];
}
**
void rk(double h, int N,
void (*dXdt)(double t, double X[], double dXdt[]),
double t, double X0[], double X[])
{
int i;
arrays for
double *d1 = vector(N),*d2 = vector(N),*d3 = vector(N);
.
*/
double *Xa = vector(N),*dX = vector(N);
dXdt(t, X0, dX);
for(i = 0; i <= N - 1; i++)
{
d1[i] = h * dX[i];
Xa[i] = X0[i] + 0.5 * d1[i];
}
dXdt(t + 0. 5 * h, Xa, dX);
for(i = 0; i <= N - 1; i++)
{
d2[i] = h * dX[i];
Xa[i] = X0[i] + 0.5 * d2[i];
}
dXdt(t + 0.5 * h, Xa, dX);
for(i = 0; i <= N-1; i++)
{
d3[i] = h * dX[i];
Xa[i] = X0[i] + d3[i];
}
dXdt(t + h, Xa, dX);
for(i = 0; i <= N - 1; i++)
X[i] = X0[i] + (d1[i] + d2[i] * 2 + d3[i] * 2 + h * dX[i]) / 6.0;
free_vector(d1); free_vector(d2); free_vector(d3);
free_vector(Xa); free_vector(dX);
}
lorenz.h
The Lorenz equations
**
** parameters, and this time, they are set to
** as follows:.
#define dxdt dXdt[0]
#define dydt dXdt[1]
#define dzdt dXdt[2]
#define x X[0]
#define y X[1]
#define z X[2]
The Lorenz equations
void lorenz(double t, double X[], double dXdt[])
{
double sigma=10.0, b=8.0 / 3, r=28.0;
dxdt = -sigma * x + sigma * y;
dydt = -x * z + r * x - y;
dzdt = x * y - b * z;
}
#undef dxdt
#undef dydt
#undef dzdt
#undef x
#undef y
#undef z
Result
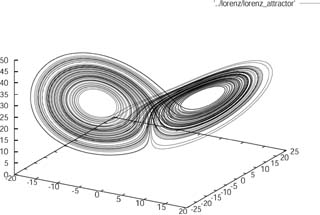
Fig. 1: The Lorenz Attractor
(PS, PDF,
PSD, PSD
(for the web), AI).
Appendix
Fig. 2 demonstrates a pteridophyte (fern) drawn by using "gnuplot ":
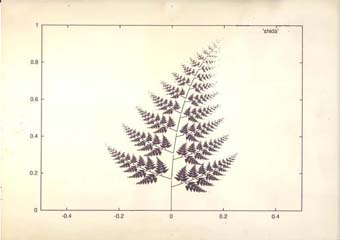
Fig. 2: A pteridophyte (fern).
| Top page |
Feedback
Message Form 
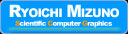
Supplementary Information: Ryoichi Mizuno - Google+ 
|